Simple Example
- Example 1
- using System;
class A
{
public A() { Console.WriteLine("Creating A"); }
~A() { Console.WriteLine("Destroying A"); }
}
- class B : A
{
public B() { Console.WriteLine("Creating B"); }
~B() { Console.WriteLine("Destroying B"); }
}
- class C : B
{
public C() { Console.WriteLine("Creating C"); }
~C() { Console.WriteLine("Destroying C"); }
}
- class App
{
public static void Main()
{
C c = new C();
Console.WriteLine("Object Created ");
Console.WriteLine("Press enter to Destroy it");
Console.ReadLine();
c = null;
Console.Read();
}
}
- result
}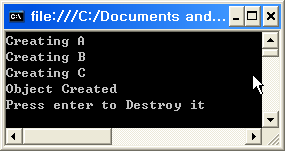
- Example 2
- using System;
class A
{
public A() { Console.WriteLine("Creating A"); }
~A() { Console.WriteLine("Destroying A"); }
}
- class B : A
{
public B() { Console.WriteLine("Creating B"); }
~B() { Console.WriteLine("Destroying B"); }
}
- class C : B
{
public C() { Console.WriteLine("Creating C"); }
~C() { Console.WriteLine("Destroying C"); }
}
- class App
{
public static void Main()
{
C c = new C();
Console.WriteLine("Object Created ");
Console.WriteLine("Press enter to Destroy it");
Console.ReadLine();
c = null;
GC.Collect();
Console.Read();
}
}
- result

- Unmaged Heap Memory:
- for C,C++ , Memeorry Alocation, insances
- cannot be reachable by GC
- use pointer
- Managed Heap Memory
- for .net,
- reachable by GC,
- no pointer => the rumtime can do memory magagenemt
Garbage Collector(GC) in .net
- concept
- Thread
- 생성된 객체중에서 접근이 불가능한 객체의 메모리를 수집하여 해제한다.
- The task of garbage collection is a costly process
- GC의 실행시기
- when a call to create a new object fails due to lack of memory
managed heap memory가 부족할 때(다른 객체의 생성이 불가능 때)
- when the user explicitly calls GC thread to do garbage collection by calling the function GC.Collect().
- GC can recollect memory from only those objects which are no longer accessible in your code by any reference
- if a function goes out of scope,
- all its local objects will be eligible for garbage collection, unless and until of course the local objects have their references passed outside the function
- In the case of the windows service,
- there might be some objects which are no longer required to be referenced but still have references pointing to them.
- So for them to be eligible to Garbage Collection I have to remove all the references to these objects programmatically
- 예1
- void A() {
B(); //1
int i = 10; //2
}
void B() {
C c = new C();
Console.WriteLine(c.Name);
}
- 실행이 문장 2에 도달하면 B()에서 생성돤 객체 c늬 접근이 불가하므로 다음 GC가 실행될때 c츼 객체는 해제된다.
- 예 2
- class A {
pribate B b; // line 3
public void RequiredB() {
b = new B(); //line 4
b.DoSomeWork();
b=null; //line 5
}
- 함수 RequiredB가 실행이 완료되어도 line2의 참조 b 때문에 객체 B가 유지된다. RequiredB()의 실행이 종료되면 참조 nuul로 참조 b를 세팅하여 객체 B를 GC가 가능하게 하였다.
- Multi Reference
- class A
{
private B b; //reference now points to nothing, trying to use this directly will give NullPointerException
private B b2; //another empty reference which points to nothing
public void RequireB()
{
b = new B(); //Set this reference to an object
b2 = b; //Also set b2 as reference to the same object pointed by b Now object created above by calling new B() has 2 references b and b2
b2.DoSomeWork(); //b2.DoSomeWork is same as b.DoSomeWork() as both point to same object
b = null; //Just doing this will not make the object eligible for garbage collection because even b2 is pointing to it. So you need the line below too! Else there will be a memory leakage
b2=null;
}
}
- Dispose, IDisposable
- B b = new B();
using (b)
{
// use b
} // here compiler will call Dispose on b's IDisposable implementation automatically
-
- class K
{
K() {}
void Finalize() //GC가 자동 호출
{
base.Finalize();
}
}
-